Photo-gate timer with CircuitPython
From ShawnReevesWiki
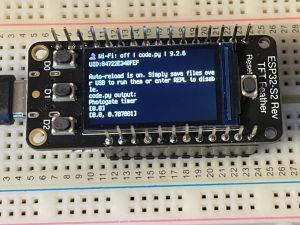
See Photo-gate timer for a full discussion.
CircuitPython
CircuitPython is a flavor or port of MicroPython, by Adafruit Industries, to program any of hundreds of micro-controllers or single-board-computers with Python code. Changing the code on a board is simple: You copy a Python program file, and optionally libraries, to board that can be set to present itself to a computer as a USB drive. When you reset the board, it runs the file.
ESP32-S2 Reverse TFT Feather
The ESP32-S2 Reverse TFT Feather from Adafruit, https://www.adafruit.com/product/5345 , is an inexpensive development board with a WiFi capable radio.
The same text output to the Python REPL on the computer is output to the screen on the module, so outputting text requires only the built-in print() function.
Code
import time
import board
import keypad
print("Photogate timer")
gates = (board.D1, board.D2)
#create empty list:
times = []
#track which gates have already been interrupted
passed = []
#interval is time in seconds to debounce button.
keys = keypad.Keys(gates, value_when_pressed=True, pull=True, interval=0.002)
while True:
event=keys.events.get()
if event:
#print(event) #uncomment this to debug events
#which gate was passed? event.key_number
#if this is the first gate:
if 0 == len(times):
times.append(0.0)
startTime = time.monotonic()
#mark which gate has been passed
passed.append(event.key_number)
print(times)
#else check if a gate not yet passed:
elif 0 == passed.count(event.key_number):
#Add the current time to the list
times.append(time.monotonic() - startTime)
passed.append(event.key_number)
print(times)